Build with Confidence
Boost your Postgres abilities with Crunchy Data
Enable your organization to build confidently with Postgres
From startups to Fortune 500s, Crunchy Data is the trusted partner organizations can rely on for their Postgres needs. From healthcare, software development, autonomous vehicles, government agencies, financial services to decentralized finance companies, all types of industries trust Crunchy Data.
Postgres anywhere you want it
Bare Metal, VMs, Cloud
Crunchy Postgres
Crunchy Certified PostgreSQL is production ready postgres for anywhere you want to run it.
What to expect:
- Backups
- Disaster recovery
- High availability
- Monitoring
- Automation
- Self managed
Kubernetes
Crunchy Postgres for Kubernetes
Cloud Native Postgres on Kubernetes powered by Crunchy Postgres Operator (PGO).
What to expect:
- Simple provisioning
- Backups and DR included
- High availability
- Seamless upgrades
- Scale from 1 to thousands of databases
- Self managed
Fully Managed Cloud
Crunchy Bridge
The fully managed Postgres option on your choice of Cloud provider.
What to expect:
- Cloud agnostic (AWS, Azure, GCP)
- Continuous protection
- Backups included
- Point in time recovery built-in
- Pay for what you use
- The developer experience you want
Enterprise Support
Crunchy Data Support included with all Crunchy Data Product subscriptions.
It's a real partnership. Night and day difference between our former Postgres support provider and Crunchy Data.Tim Gombold
Director of IT Infrastructure, Foundever®
FoundeverCrunchy Postgres
Learn how always on Crunchy Postgres helped the team at Foundever sleep at night.
Read Case StudyPostgres is the right choice for your data modernization.
Everyone from start-ups to enterprises are choosing Postgres for innovation. Find out why Postgres is one of the most advanced and loved open source databases.
Production Ready
Crunchy Postgres is production ready Postgres
Companies of all sizes, of any enterprise or industry, can benefit from moving operations to PostgreSQL. We can help you with your journey and assist in providing the operational and developer experience you've always wanted with the database you've always loved.
Backups
- Data is one of your most valuable assets, Crunchy Postgres ensures your data is safe and backed up so you can sleep easy.
Disaster recovery
- When the worst happens we have you covered. Never worry about data corruption or loss with point-in-time recovery.
High availability
- With high availability you can trust your database is online.
Connection scaling
- With built in connection scaling you can easily scale to tens of thousands of connections for your database.
Monitoring
- Get the insights you need to know what is happening with your database with monitoring included.
No lock-in
- With Crunchy Data and open-source Postgres you are not locked-in to proprietary technology.
Crunchy Bridge
Cloud Postgres built for a superior developer experience
On Crunchy Bridge, Developer Experience (DX) means a toolset that gets you into the zone. Our platform has the tools necessary for developers to execute the optimum decision for performance and scale today while looking into the future.
“The Crunchy team is awesome to work with, very responsive, very smart, and are clearly committed to getting the customer issues solved. We are working with Crunchy for the people and the technology.”
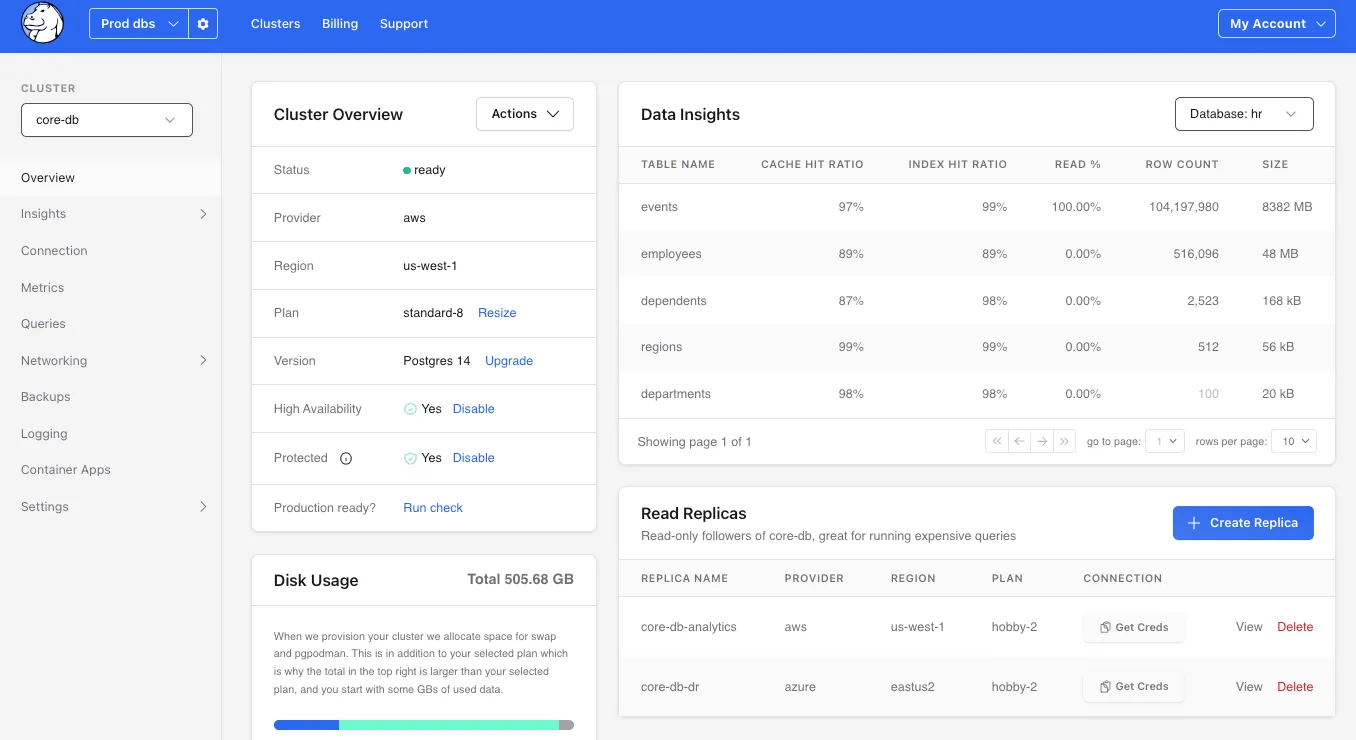